Import Maps Everywhere
Javascript import maps provide a mapping between local names and external modules.
Using Import Maps is a two-step process. First, you create a script with a type="importmap"
attribute to create the mappings.
<script type="importmap">
{
"imports": {
"browser-fs-access": "https://unpkg.com/browser-fs-access@0.33.0/dist/index.modern.js"
}
}
</script>
You can also reference files in the project's node_modules folder.
<script type="importmap">
{
"imports": {
"lodash": "/node_modules/lodash-es/lodash.js"
}
}
</script>
Furthermore, we can map multiple modules inside the path that we're mapping to.
For example, lodash has many modules living under the lodash module. We can use a slash after the map to indicate that we also want the content inside the module.
<script type="importmaps">
{
"imports": {
"lodash": "/node_modules/lodash/lodash.js",
"lodash/": "/node_modules/lodash/"
}
}
</script>
This enables us to import the full package directly.
import _lodash from "lodash";
Or to import a component module from the package.
import _shuffle from "lodash/shuffle.js";
The second step is to use the module we specified in the import map on our scripts by referencing the mapped specifier rather than the URL.
<button>Select a text file</button>
<script type="module">
import { fileOpen } from 'browser-fs-access';
const button = document.querySelector('button');
button.addEventListener('click', async () => {
const file = await fileOpen({
mimeTypes: ['text/plain'],
});
console.log(await file.text());
});
</script>
This is the basic usage of import maps. The specification README outlines additional uses for the API, including scoping the imports.
The important element (and why I revisited the API now) is that the API is now supported in all browsers so it's worth revisiting it and testing if suits your needs.
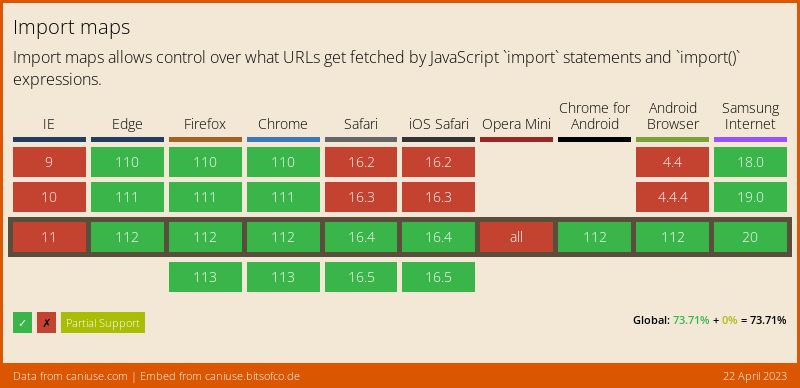